Scss/flocssで自作ユーティリティー border編
u-b-grayやu-bt-primary-1など「u-b」の接頭辞がついた、ユーティリティーを作成します
フォルダ構成やutilityの適用順などはflocssを基準にしています
社内のコーディング共通フォーマットとして少しずつ独自の改良をしてきた設定です
下記もあわせてご覧ください
- Scss/floccsで自作ユーティリティー margin / padding編
- Scss/floccsで自作ユーティリティー display / gap編
- Scss/floccsで自作ユーティリティー font-size / font-weight編
- Scss/floccsで自作ユーティリティー border編
- Scss/floccsで自作ユーティリティー width編
- Scss/floccsで自作ユーティリティー color / background-color編
ゴール
- 全体及び各辺のボーダーを設定できること
- ボーダーサイズ及びボーダーカラーの設定ができること
- border: noneでは、media queryのサイズによる階層的な適用が確実にされること
前提
foundation/variable
使用しないbreak pointを入れておくと無駄な設定ができてしまいますので、注意してください
$break-points: (
all: '',
lg: 'screen and (max-width: 1024px)',
md: 'screen and (max-width: 820px)',
) !default;
$colors: (
primary: #F48C40,
danger: #E88E7D,
info: #00B187,
secondary: #E88E7D,
success: #00B187,
warning: #ffc107,
black: #000000,
white: #ffffff,
gray: #CCCCCC,
);
foundation/mixin
@use 'sass:map';
@mixin mq($breakpoint: md) {
@if($breakpoint == 'all') {
@content;
} @else {
@media #{map.get($break_points, $breakpoint)} {
@content;
}
}
}
使用法
全囲い
.u-b-{$colors}
.u-b-{$colors}-{サイズ}
一部
.u-{方向種類}-{$colors}
.u-{方向種類}-{$colors}-{サイズ}
非表示
.u-b-none
.u-b-{$break-points}-none
パラメータ
{サイズ} … 2~10(px相当)、指定ない場合1px
{方向種類} … bt: top / br: right / bb: bottom / bl: left
{color名}は、上記前提を参照
使用例
<div class="u-b-primary u-b-lg-none">
・・・
</div>
上記では、画面幅により下記のようになります
- 1025px以上:「u-b-primary」で色が#F48C40で、1pxのボーダーで囲われます
- 821~1024px:「u-b-lg-none」でborderは非表示になります
- 820px以下:上記継承してそのまま非表示です
下記で、色名と色は自由に設定できます
$colors: (
primary: #F48C40,
danger: #E88E7D,
info: #00B187,
secondary: #E88E7D,
success: #00B187,
warning: #ffc107,
black: #000000,
white: #ffffff,
gray: #CCCCCC,
);
最終形
上記の前提は、各環境にあわせ別ファイルとして振り分け、下記ファイルで読み込んでください
@use '../../foundation/variable' as *;
@use '../../foundation/mixin' as *;
// -----------------------------------------------------------------
// Border
// -----------------------------------------------------------------
$array: (b: '', bt: 'top', br: 'right', bb: 'bottom', bl: 'left');
@each $key, $dir in $array {
.u-#{$key} {
@if $key == 'b' {
// 囲み
@each $name, $color in $colors {
&-#{""+$name} {
@for $i from 1 through 10 {
@if $i==1 {
border: 1px solid $color !important;
} @else {
&-#{$i} {
border: #{$i}px solid $color !important;
}
}
}
}
}
} @else {
// 一部
@each $name, $color in $colors {
&-#{""+$name} {
@for $i from 1 through 10 {
@if $i==1 {
border-#{$dir}: 1px solid $color !important;
} @else {
&-#{$i} {
border-#{$dir}: #{$i}px solid $color !important;
}
}
}
}
}
}
}
}
.u-b-none {
// 削除
@each $breakpoint, $query in $break-points {
@include mq($breakpoint) {
@if $breakpoint=='all' {
border: none !important;
} @else {
&-#{$breakpoint} {
border: none !important;
}
}
}
}
}
解説
上記最終系を一つずつ見ていきます
ループ設定
$array: (b: '', bt: 'top', br: 'right', bb: 'bottom', bl: 'left');
上記$arrayをループして展開していきます
@each $key, $dir in $array {
.u-#{$key} {
@if $key == 'b' {
・・・
} @else {
・・・
}
}
}
$keyが「b」の場合とそれ以外で設定を変えています
展開されるイメージは下記の通りです
.u-b {}
.u-bt {}
.u-br {}
・・・
詳細クラスの設定
ボーダー
@each $key, $dir in $array {
.u-#{$key} {
@if $key == 'b' {
// 囲み
@each $name, $color in $colors {
&-#{""+$name} {
@for $i from 1 through 10 {
@if $i==1 {
border: 1px solid $color !important;
} @else {
&-#{$i} {
border: #{$i}px solid $color !important;
}
}
}
}
}
} @else {
// 一部
@each $name, $color in $colors {
&-#{""+$name} {
@for $i from 1 through 10 {
@if $i==1 {
border-#{$dir}: 1px solid $color !important;
} @else {
&-#{$i} {
border-#{$dir}: #{$i}px solid $color !important;
}
}
}
}
}
}
}
}
上記ブロックの真ん中の部分を解説していきます
「b」の場合 / 全囲い
// 囲み
@each $name, $color in $colors {
&-#{""+$name} {
@for $i from 1 through 10 {
@if $i==1 {
border: 1px solid $color !important;
} @else {
&-#{$i} {
border: #{$i}px solid $color !important;
}
}
}
}
}
全囲いの設定となります
@each $name, $color in $colors {
&-#{""+$name} {
上記で下記の各色設定を展開し、「.u-b-primary」のようにclassを作成しています
$colors: (
primary: #F48C40,
danger: #E88E7D,
info: #00B187,
secondary: #E88E7D,
success: #00B187,
warning: #ffc107,
black: #000000,
white: #ffffff,
gray: #CCCCCC,
);
下記で1pxをデフォルトとして10pxまでの幅に対応できるclassを作成しています
@for $i from 1 through 10 {
@if $i==1 {
border: 1px solid $color !important;
} @else {
&-#{$i} {
border: #{$i}px solid $color !important;
}
}
}
下記が展開イメージとなります
.u-b-primary {
border: 1px solid #F48C40 !important;
}
.u-b-primary-2 {
border: 2px solid #F48C40 !important;
}
.u-b-primary-3 {
border: 3px solid #F48C40 !important;
}
・・・
.u-b-gray {
border: 1px solid #CCCCCC !important;
}
.u-b-gray-2 {
border: 2px solid #CCCCCC !important;
}
.u-b-gray-3 {
border: 3px solid #CCCCCC !important;
}
・・・
「b」以外の場合 / 一部
各辺の設定になります
下記が展開イメージとなります
.u-b-bt-primary {
border-top: 1px solid #F48C40 !important;
}
.u-b-bt-primary-2 {
border-top: 2px solid #F48C40 !important;
}
.u-b-bt-primary-3 {
border-top: 3px solid #F48C40 !important;
}
・・・
.u-b-bt-gray {
border-top: 1px solid #CCCCCC !important;
}
.u-b-bt-gray-2 {
border-top: 2px solid #CCCCCC !important;
}
.u-b-bt-gray-3 {
border-top: 3px solid #CCCCCC !important;
}
・・・
非表示
ボーダーをすべて非表示にする設定です
$break-points: (
all: '',
lg: 'screen and (max-width: 1024px)',
md: 'screen and (max-width: 820px)',
) !default;
上記$break-pointsをループしてborder: none設定をしています
.u-b-none {
// 削除
@each $breakpoint, $query in $break-points {
@include mq($breakpoint) {
@if $breakpoint=='all' {
border: none !important;
} @else {
&-#{$breakpoint} {
border: none !important;
}
}
}
}
}
下記が展開イメージとなります
.u-b-none {
border: none
}
.u-b-none-lg {
border: none
}
.u-b-none-md {
border: none
}
まとめ
以上でborderのユーティリティーの完成となります
全設定が吐き出されてしまうと膨大な量になってしまいますが、弊社では、vite+@fullhuman/postcss-purgecssなどを使って、余計なclassは入らないようにしていますので、そういった工夫も必要になるかと思います
コーディングの省力化に貢献できれば幸いです
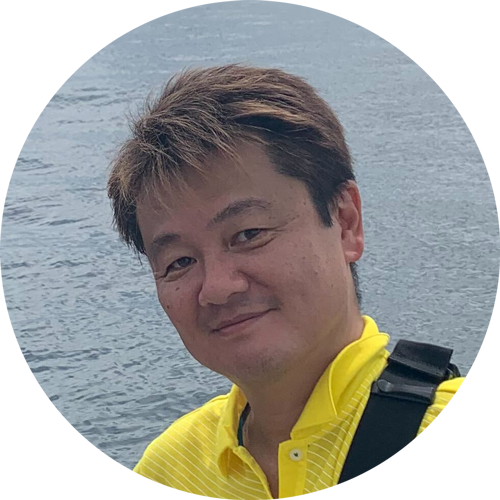
文責:フライング・ハイ・ワークス代表 松田 治人(まつだ はるひと)
会社では、Laravelを中心としたエンジニアとして働いており、これまでに50本以上のLaravelによる東京でWebシステムの制作やホームページ制作をしています。
エンジニアとして弊社で働きたい方、お仕事のご相談など、お待ちしております。
WEBサイト・ホームページの制作をご検討の方
フライング・ハイ・ワークスの紹介
フライング・ハイ・ワークスは、東京のホームページ制作・Web制作会社・Webシステム制作会社です。東京都及びその近郊(首都圏)を中心として、SEO対策を意識したPC及びスマホのサイトをワンソース(レスポンシブ対応)で制作します。
実績
デザイナーチームは、グラフィックデザインやイラストの制作も得意としており、著作権を意識しない素材の提供が可能です。システム・コーディングチームでは、Laravelなどを使用したスクラッチからのオリジナルシステム開発を始め、WordPressのカスタマイズを得意としております。
また、SEOやランディングページ(LP)、広告向けバナーなどを他社様でやっていた作業の引継ぎでも問題ありません。制作実績は多数ございますので、お客様に合わせたご提案が可能です。
500点以上のフライング・ハイ・ワークスの制作実績ページをご覧ください。
ホームページ制作・Web制作のお問い合わせ、お見積り依頼、相談、質問は
お問い合わせフォームよりお願いいたします